驾驶员状态检测
CarND-课程概览
无人驾驶课程一共有三个学期,每个学期三个月。
新手大礼包:CarND-Term1-Starter-Kit
测试环境:CarND-Term1-Starter-Kit-Test
第一学期
第一学期主要介绍了图像方面的基础知识和应用,包括传统数字图像处理技术和深度学习。
P1 检测车道线
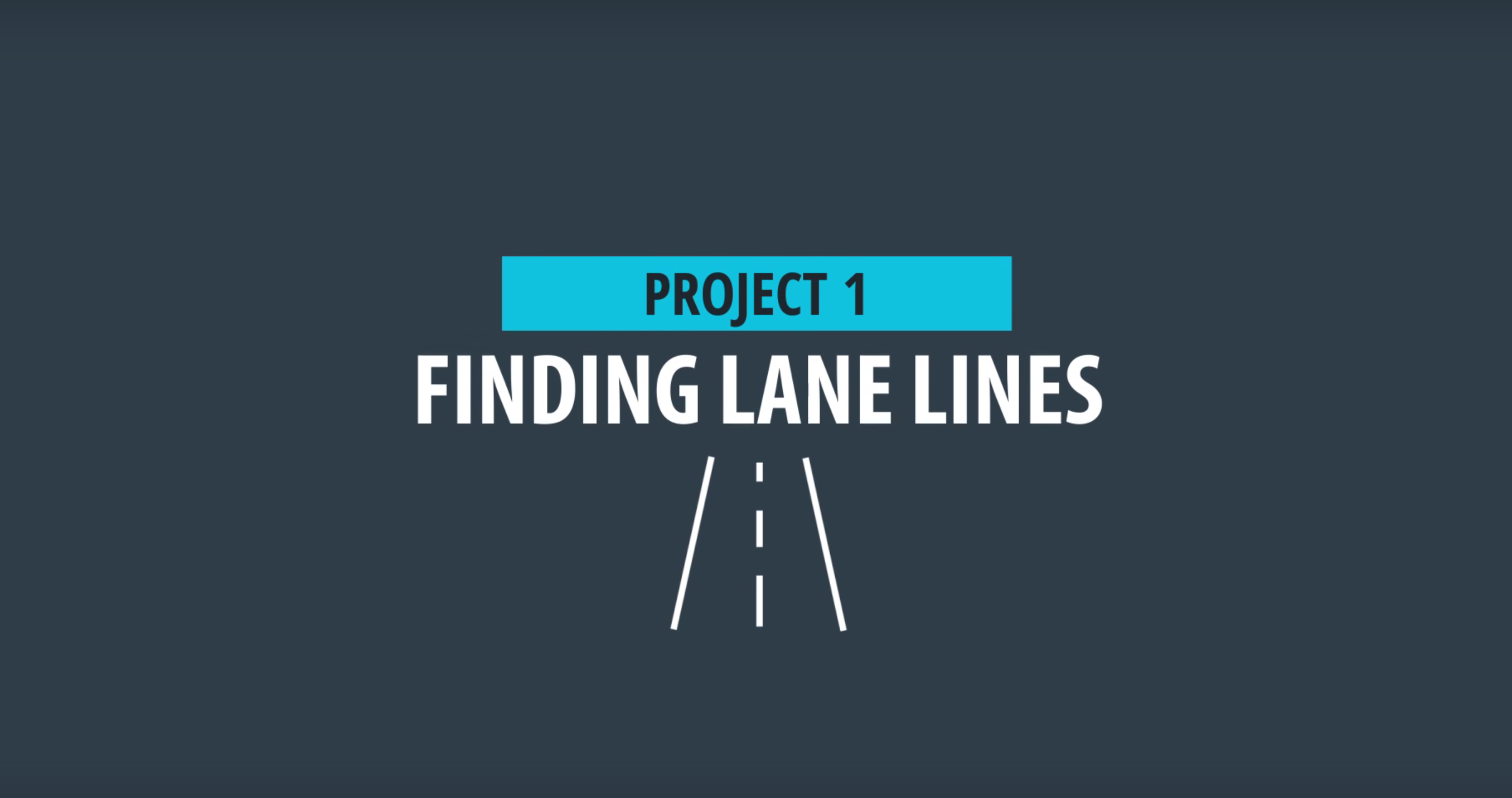
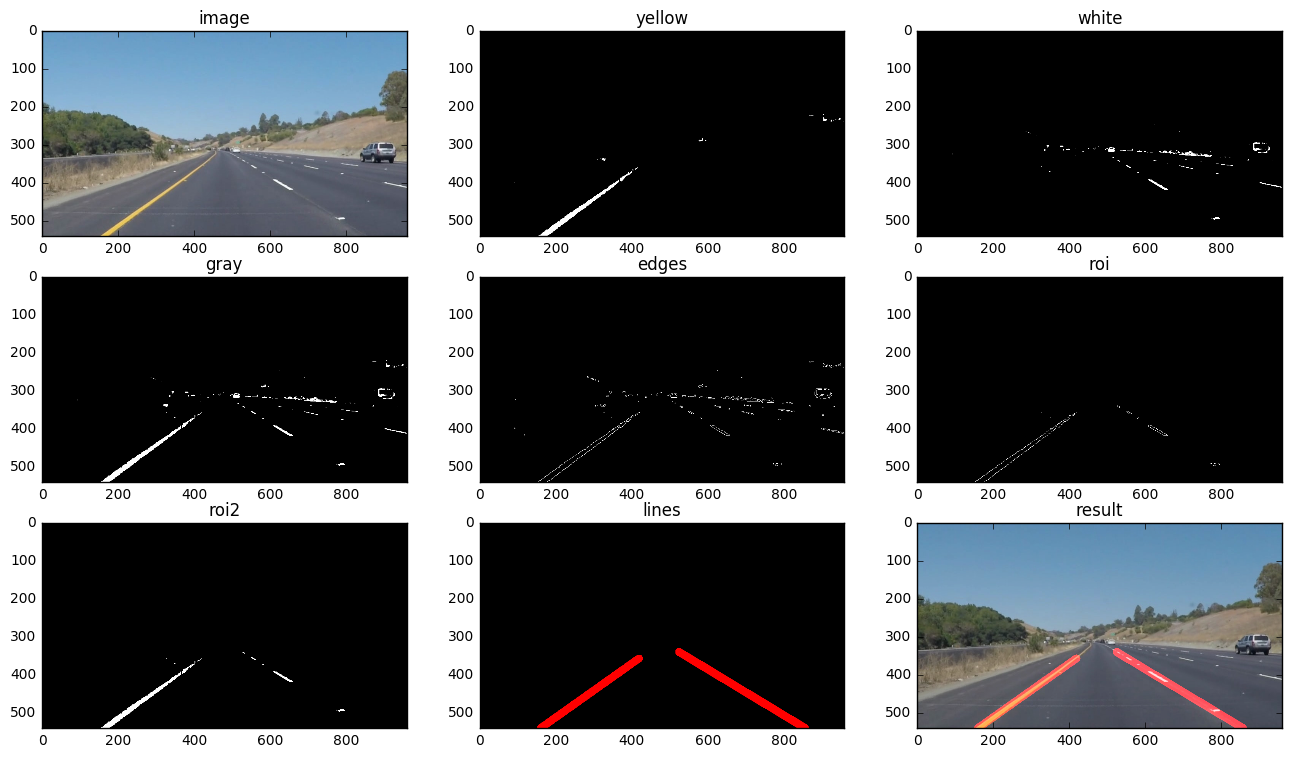
使用 Karabiner 自动区分罗技鼠标和触控板
1 | <?xml version="1.0"?> |
在 Misc & Uninstall 中 Open private.xml ,然后将上面的代码粘贴,在 Change Key 中 Reload XML ,勾选罗技鼠标反转,即可自动区分鼠标与触控板,触控板保持自然方向,鼠标保持原来的滚动方向。
python 改豆瓣源
1 | mkdir ~/.pip/ |
Linux service启动项
首先我们需要将启动的命令写入/etc/init.d/目录下: 1
2
3sudo nano /etc/init.d/ypwtest
/usr/bin/aria2c --enable-rpc --rpc-listen-all=true --rpc-allow-origin-all1
update-rc.d ypwtest defaults 99
1
update-rc.d -f ypwtest remove
三种方式控制NanoPi2的GPIO
首先奉上PDF资料,万变不离其宗,掌握核心科技才是最重要的:
SEC_Users_Manual_S5P4418_Users_Manual_Preliminary_Ver.0.10.pdf
我目前找到了三种方式控制NanoPi2的IO口:
- 通过sysfs(/sys/class/gpio)来操作;
- 通过内核的gpio_set_value函数来操作;
- 通过配置寄存器(0xC001X000)来操作。
NanoPi2内核驱动
makefile:
1 | obj-m:=gpio.o |
1 | #include <linux/kernel.h> |
使用NanoPi2的串口
今天我们目的是实现串口回环测试,意思就是自己给自己发,然后自己接收到自己发送的内容。
首先呢,根据pinMap我们可以知道,8脚是UART3_TXD,10脚是UART3_RXD,所以我们需要将NanoPi2的8脚和10脚用杜邦线接起来。
然后呢,我们连接NanoPi2,写一个程序循环读取串口数据,并打印到屏幕上。
1 | #include <stdio.h> |
1 | nano read.c |
然后我们向串口写入数据:
1 | echo hello > /dev/ttyAMA2 |
read程序就会输出刚才发送的字符,带换行。
让NanoPi2支持USB声卡
首先必须修改内核,因为目前官方默认的内核是不带USB声卡驱动的。修改内核就必须先编译uboot中的mkimage。所以先编译mkimage:
1
2
3
4
5
6
7sudo apt-get install git
git clone https://github.com/friendlyarm/uboot_nanopi2.git
cd uboot_nanopi2
git checkout nanopi2-lollipop-mr1
make CROSS_COMPILE=arm-linux- tools
sudo mkdir -p /usr/local/sbin && sudo cp -v tools/mkimage /usr/local/sbin
然后下载内核源码并开始配置: 1
2
3
4
5git clone https://github.com/friendlyarm/linux-3.4.y.git
cd linux-3.4.y
git checkout nanopi2-lollipop-mr1
make nanopi2_linux_defconfig
make menuconfig1
2
3
4Device Drivers -->
Sound card support -->
Advanced Linux Sound Architecture -->
USB sound devices
然后保存配置,编译uImage: 1
2touch .scmversion
make uImage1
scp /home/ypw/Desktop/linux-3.4.y/arch/arm/boot/uImage root@192.168.2.22:/media/fa/boot2/uImage.hdmi
注意:此处由于我并没有接LCD,所以我直接覆盖的hdmi内核,需要接LCD的话,还需要这样修改内核:
1
2
3
4
5
6make menuconfig
Device Drivers -->
Graphics support -->
Nexell Graphics -->
[*] LCD
[ ] HDMI1
2
3scp /Users/ypw/Desktop/周杰伦\ -\ 青花瓷.mp3 root@192.168.2.22:/home/fa/Desktop/qinghuaci.mp3
ssh root@192.168.2.22
mplayer /home/fa/Desktop/qinghuaci.mp3
当然,也可以在vnc中播放音乐